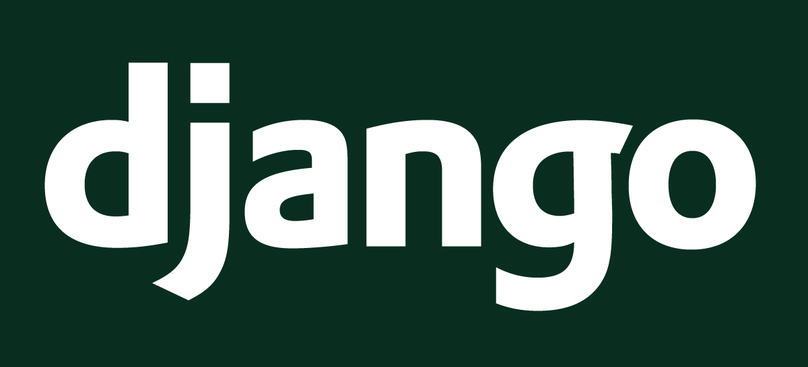
Django
Due Date |
---|
Introduction
So After AJAX, we are left with Web Backend to gain a good command over web dev. That is why we bring you here, to Django!
Django is a Loosely Coupled Framework, that uses MVC (Model-View-Controller) core architecture. What is MVC, well that's not important for now.
Here is a brief intro to why we use Django:

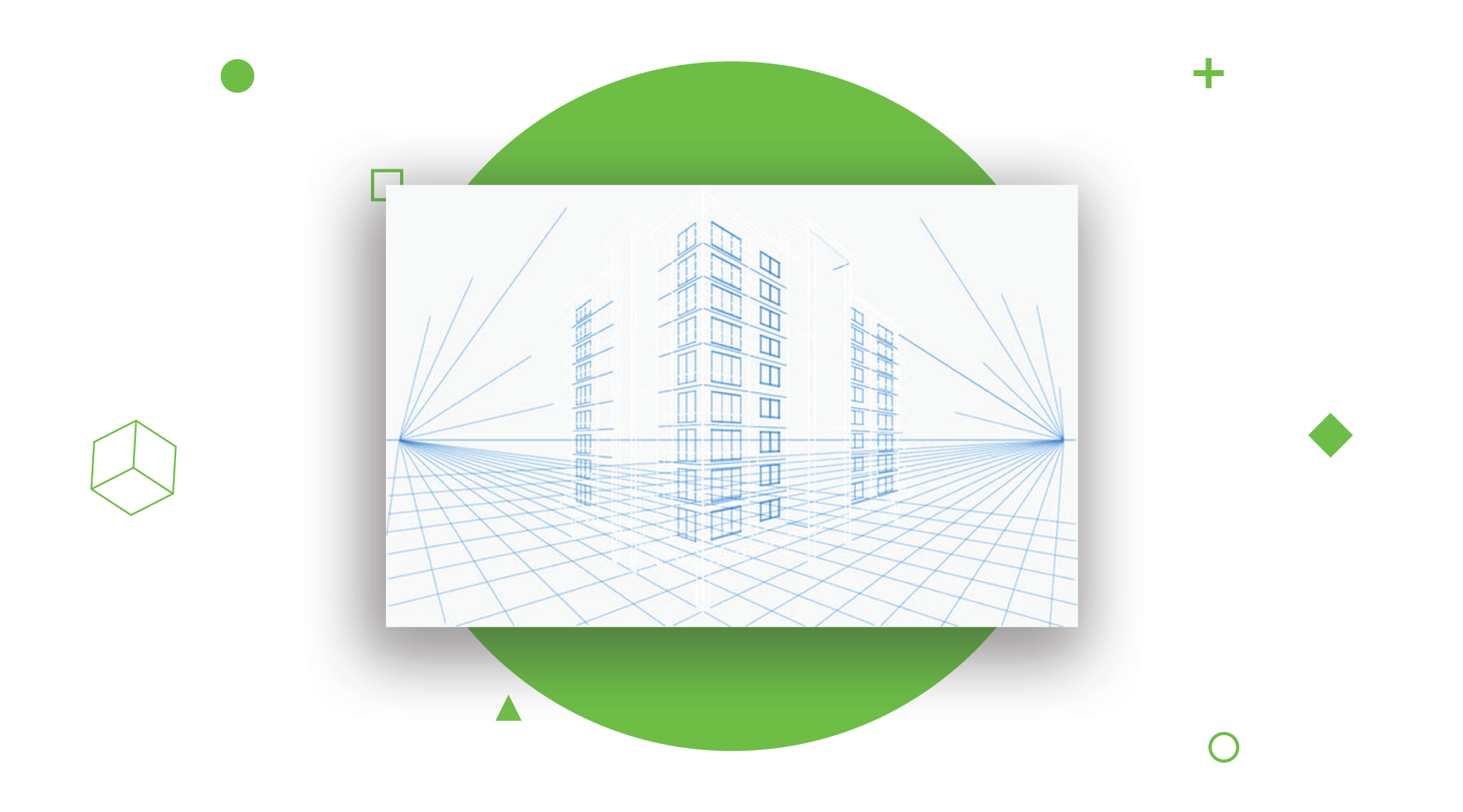
Check out the official site:
Why was Django made?
The backend developers needed a common interface to be able to work with seemingly different data sources and database software.
The design teams needed to manage the user experience with the tools they already had (HTML, CSS, JavaScript etc.)
The hard-core coders needed a framework that allowed them to rapidly deploy changes within the system that kept everyone happy.
Features
Django has
- Simple syntax
- Its own web server to test locally
- MVC (Model-View-Controller) core architecture
- Django comes with all the essentials needed for solving common cases
- HTTP libraries;
- Middleware support
- Great community support
- Lots of libraries available to extend the functionality
Principles
Most important principles to remember:
- DRY aka Don't Repeat Yourself
- Loose Coupling
- Explicit is better than implicit
- EAFP aka Easier to Ask Forgiveness than Permission (read more)
- Efficient SQL Queries
Resources
MVC (Model-View-Controller) core architecture

Basic
Here is a complete tutorial in Django


Alternatively, another popular Django learning site is Django Girls.
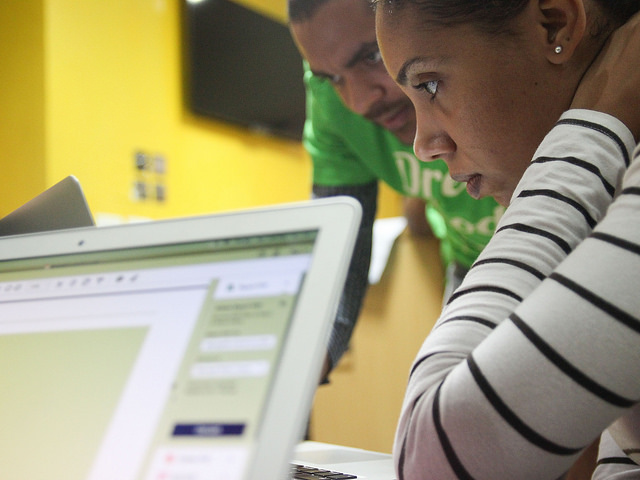
With so much serious content, you could also do well with something less technical. Here you go!
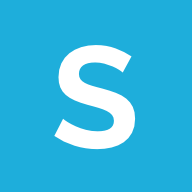
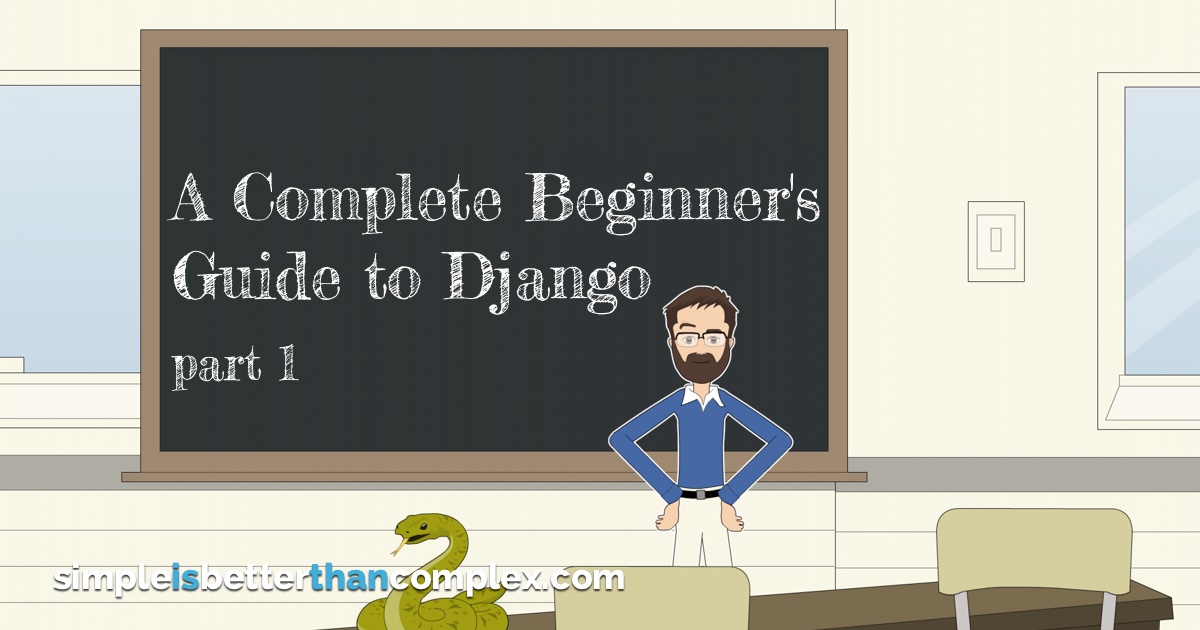
Being just introduced to Django, it would be of help to get tips from time tested users of Django.
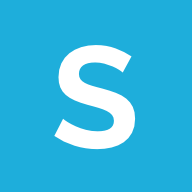
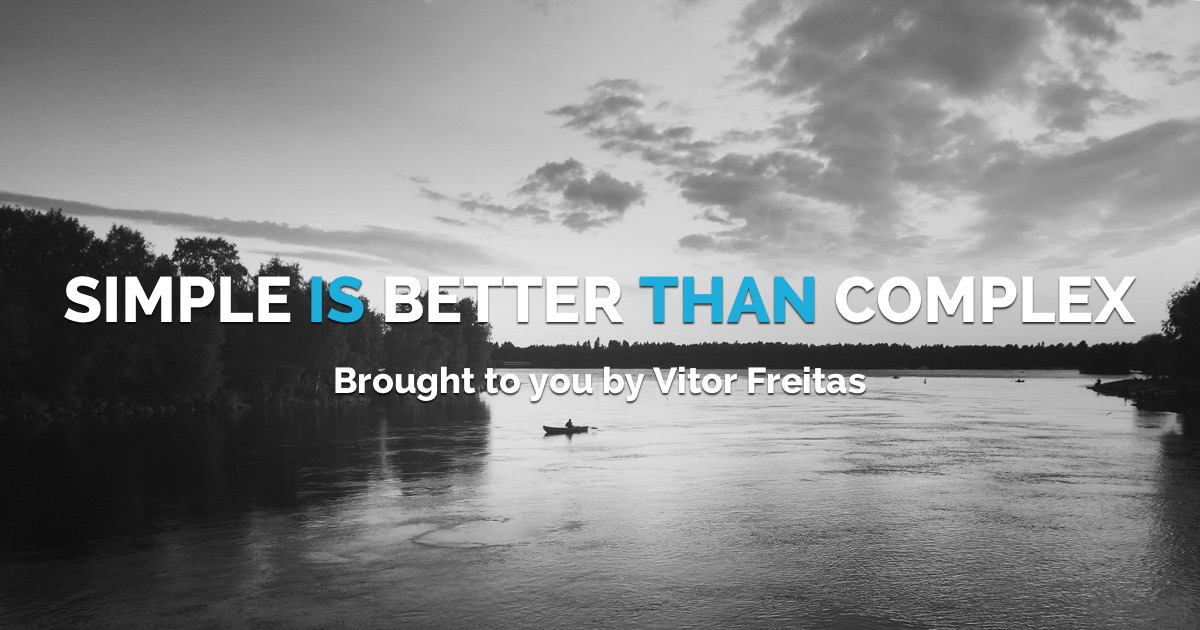
User Authentication System
Creating a backend without user login and authentication is incomplete. A user authentication system makes the app personalised for users. It takes the web app to altogether another level. Django has a built in authentication system,getting your work done with almost no effort, giving you most of the joy.
Here you can learn more about it:
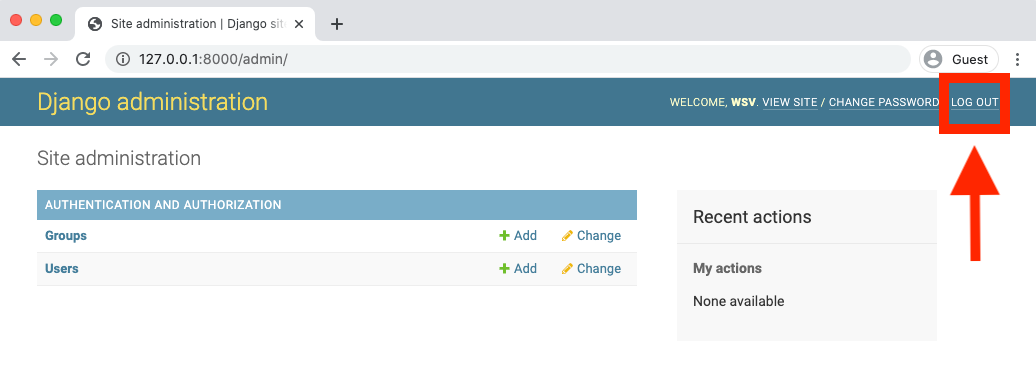
For Custom Login UI, try out this:
Challenge and Submission
So much for learning, here is your next Assignment!
All the details of the task are provided in the README.md file.
As you are aware, you need to fork from and the repository, clone the forked repository, complete the task, commit and push your changes and finally open the pull request back here.